Display
Writing Text To The Screen
- Aaron Christophel used a rect based implementation for writing characters to the screen
- This involved working through the font, writing rects (squares) everywhere there is a pixel
- This was fast enough for most text displaying needs, however it was noticeably slow when writing a full screen of text
- One factor of the slow speed was to do with the fact that the whole write into display RAM sequence was run 5*8=40 times per character
- My implementation does the work before writing, writing pixel data into the LCD Buffer directly, then using EasyDMA to write that whole buffer into display RAM
- This is different to Aarons, which draws a rect for each pixel
- This implementation is faster still than Aarons implementation, and by the naked eye, writing a full screen of text is equally as fast as drawing a rect equal to the display size (theoretically the fastest you could write to the display)
- As an aside, writing a full 240*240 rect to the display would take approximately (1/8000000*16*240*240) seconds, or about 0.1 seconds
- There are still further optimizations that could be made to this routine (and parameterising it for use with different fonts), however I am happy with the current implementation
Speeding It Up
- ATCWatch’s implementation was very inefficient in that it drew a rectangle for each pixel of the font
- This meant the display RAM write window was moved FONT_WIDTH*FONT_HEIGHT times per character, so was quite slow
My implementation is described here:
void drawCharPixelToBuffer(int charColumn, int charRow, uint8_t pixelsPerPixel, bool pixelInCharHere, uint16_t colour) {
//Get the row and column of the origin of the current FONT pixel as a DISPLAY position
//by scaling by the font size
int columnFontIndexScaledByPixelCount = charColumn * pixelsPerPixel;
int rowFontIndexScaledByPixelCount = charRow * pixelsPerPixel;
//Get the number of display pixels per character row
int pixelsPerRow = FONT_WIDTH * pixelsPerPixel;
int newXAddOffset, newYAddOffset;
//Loop through every display pixel in the current FONT pixel
for (int i = 0; i < pixelsPerPixel; i++) {
for (int j = 0; j < pixelsPerPixel; j++) {
//Handle the current offsets from the origin calculated above
newXAddOffset = columnFontIndexScaledByPixelCount + j;
newYAddOffset = rowFontIndexScaledByPixelCount + i;
//Write to the LCD buffer using the logic presented above
lcdBuffer[2*(newYAddOffset * pixelsPerRow + newXAddOffset)] = pixelInCharHere ? (colour >> 8) & 0xFF : 0x00;
lcdBuffer[2*(newYAddOffset * pixelsPerRow + newXAddOffset) + 1] = pixelInCharHere ? colour & 0xFF : 0x00;
}
}
}
- Writing a full page of size 2 font is as fast as clearing the display :)
___
Drawing Rectangle Outlines with Text
- As part of the display driver, I implemented some helper functions which would draw unfilled rectangles to the display
- I also added the functionality to put a character in the centre of that rectangle, so that you could have a sort of basic button
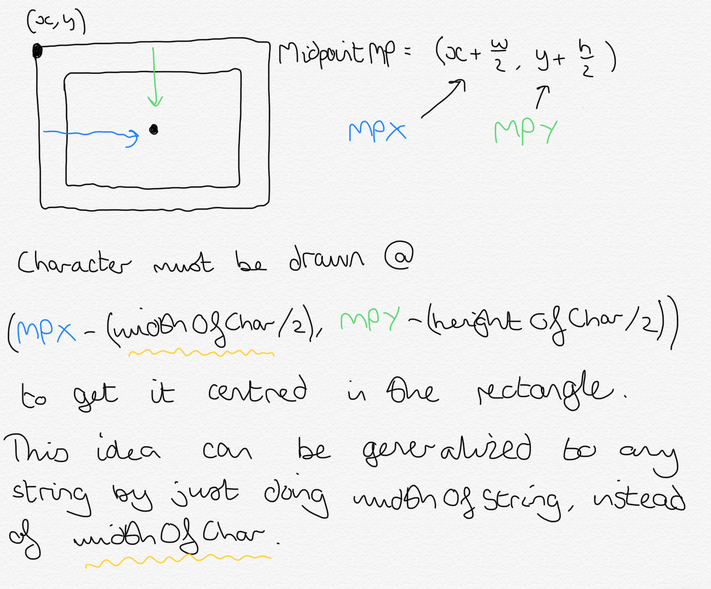